Connect an app to Attio through OAuth
Learn how to create an OAuth 2.0 app to access Attio’s REST API
If you’re building an app that needs to make requests to Attio’s REST API on behalf of many users, you should use OAuth 2.0 to authenticate your app.
This tutorial will walk you through the process of modifying an example Node.js app to connect to Attio through OAuth. We’ll start with a boilerplate app and modify it step-by-step to support generating an OAuth token for each user and then use that token to display a list of tasks they have in Attio.
Create a new app in the developer dashboard
Head over to our Developer dashboard and sign in with your Attio account.
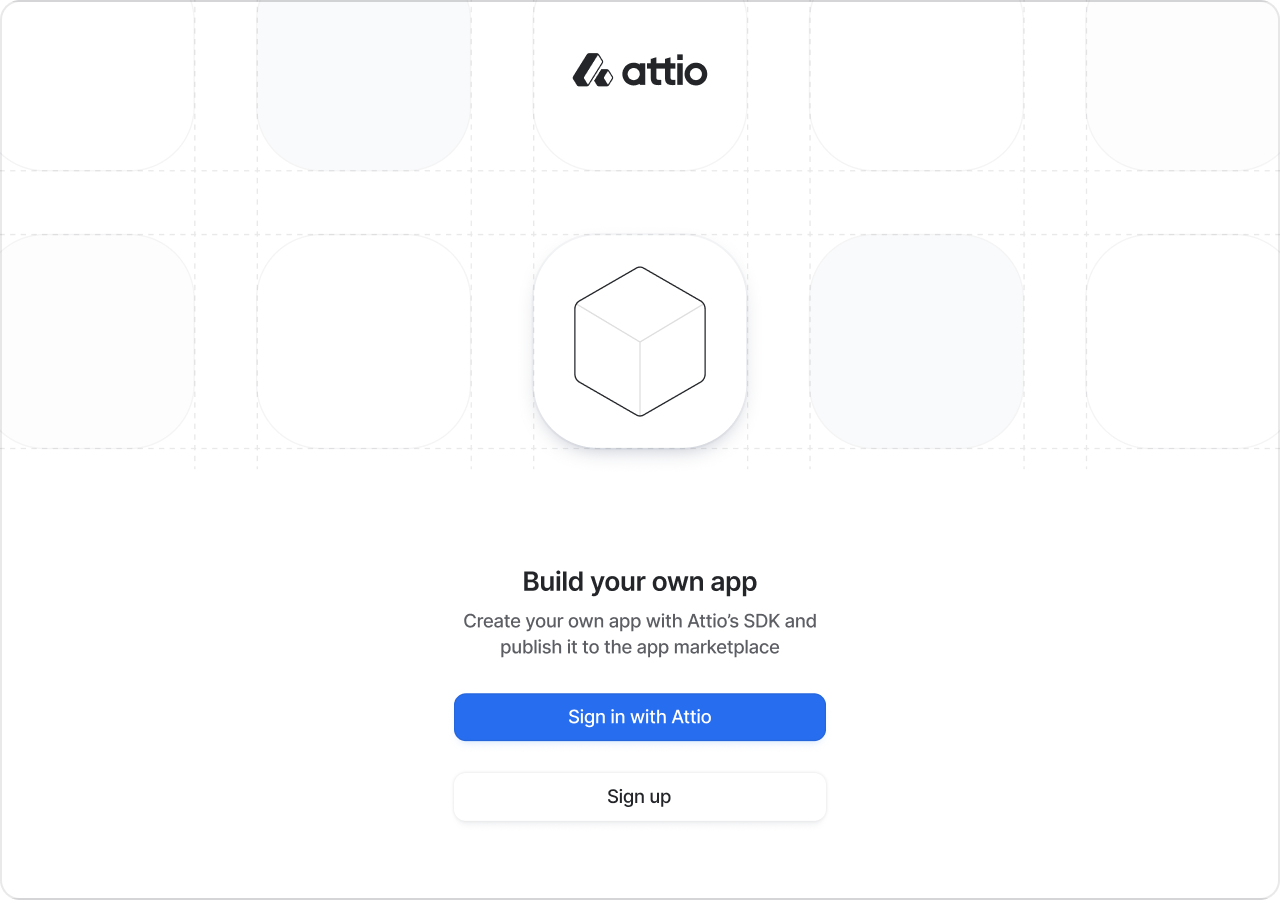
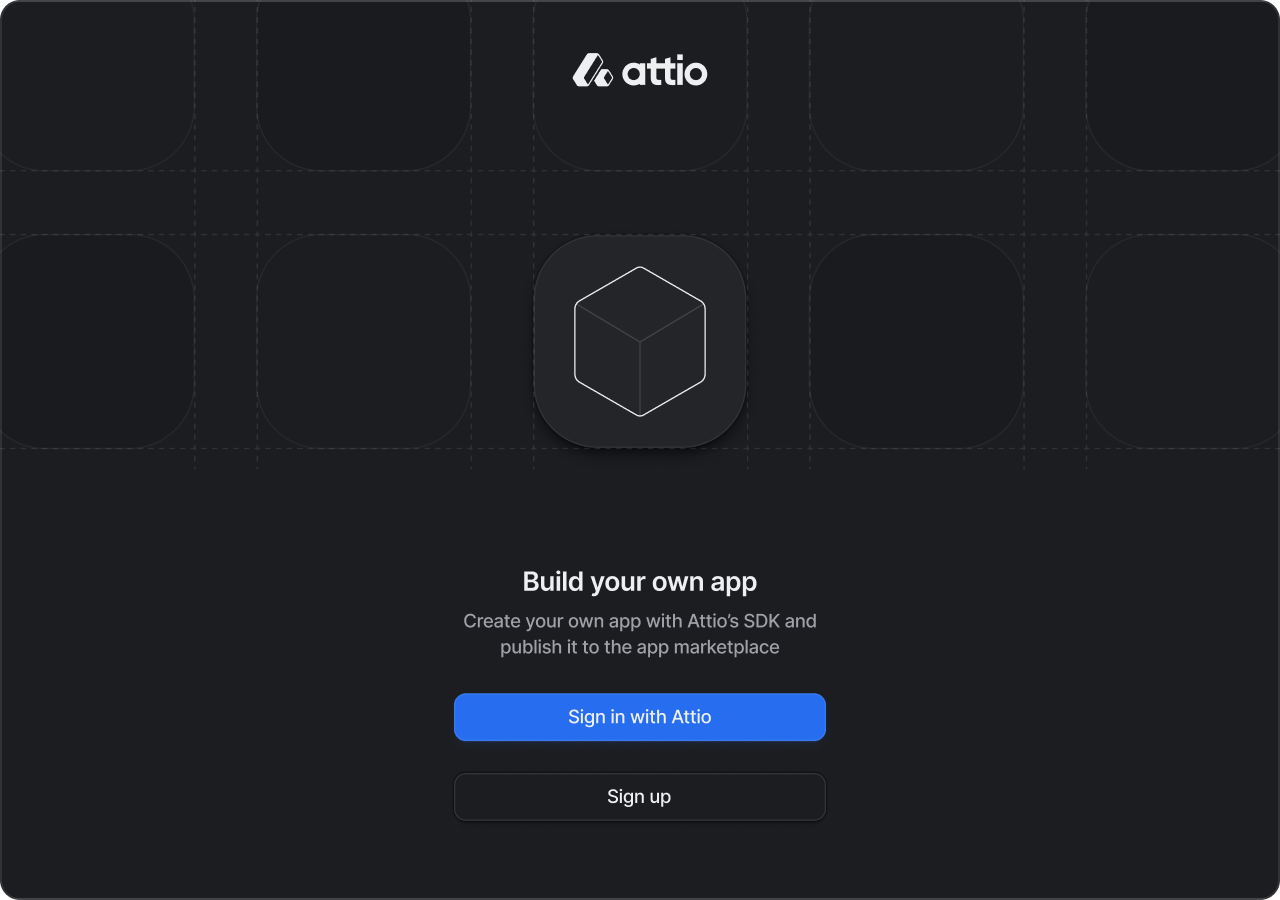
Then, create a developer account. You should set the name of your account to the name of your company or organization.
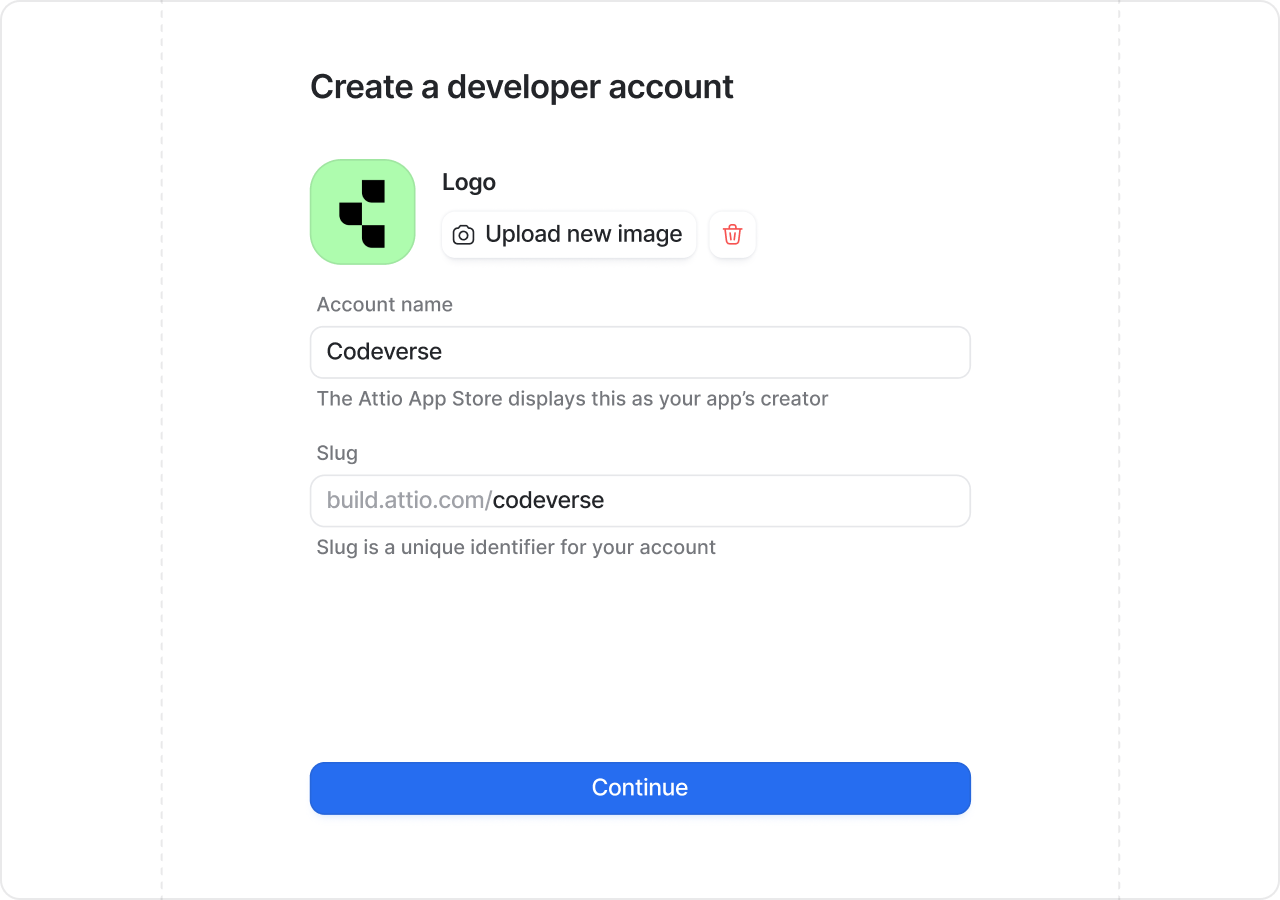
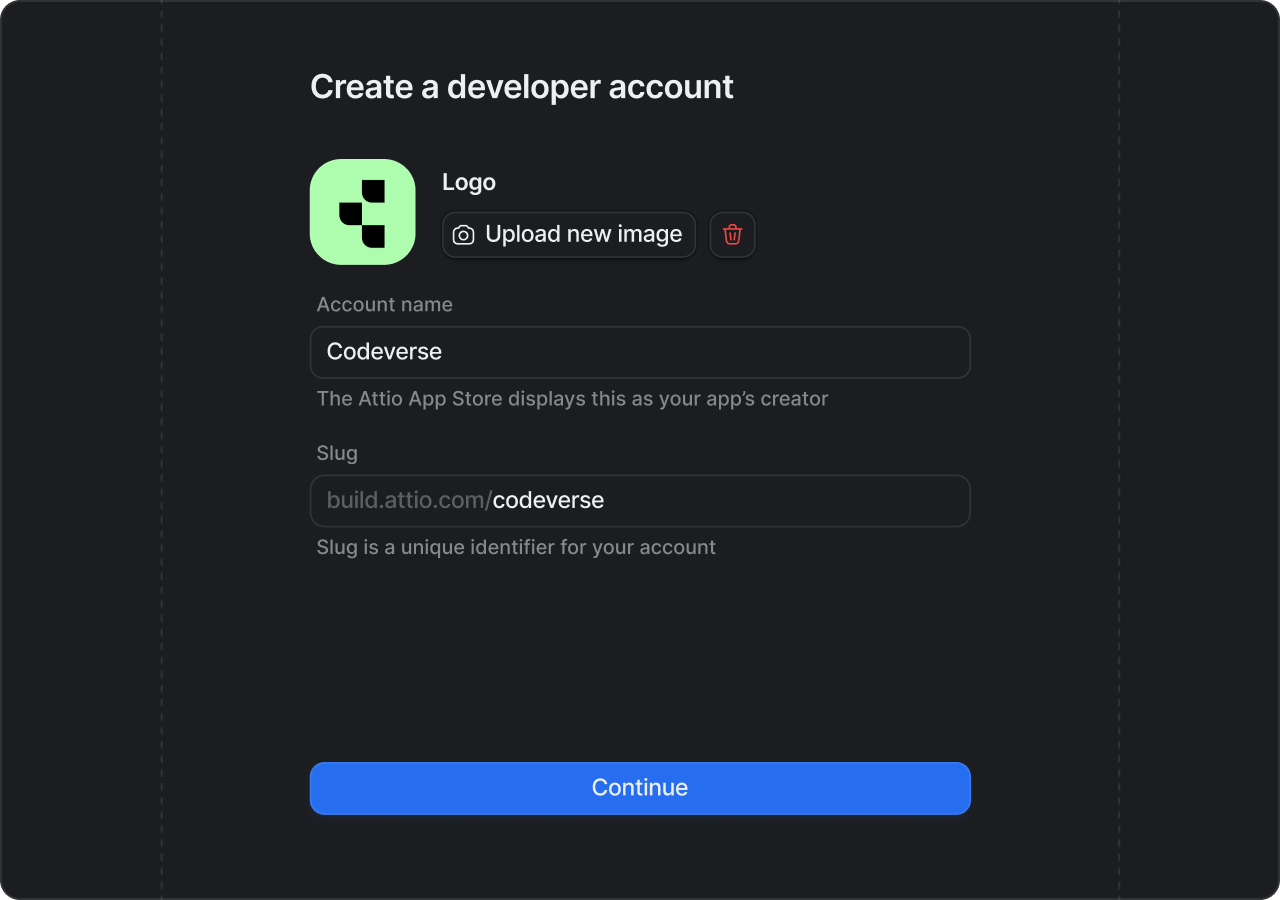
Once you’ve created a developer account, you’ll be able to create an app. Give it a unique name and hit create.
Configure OAuth
Our next job is to enable OAuth 2.0 for the app. Head to the OAuth tab in your app’s settings and enable OAuth 2.0 via the toggle at the top of the page.
Next, configure the redirect URIs for your app. For our tutorial, we’ll use the following URL:
Of course, for a real app, you’d also include a publicly available URL such as
https://my-app.com/integrations/attio/callback
.
Lastly, we need to configure the app’s scopes. Heads to the scopes tab to enable these. For our demonstration app, we’ll set tasks, user management, object configuration and records to “read” so we can fetch a list of tasks and which users they are assigned to.
Setup the Node.js project
Make a new directory and setup your new Node.js project inside it:
Create a new file called server.js
and add the following code:
You should now be able to run your app from the command line and visit it in your browser at
http://localhost:3050
:
Run through the signup flow to ensure everything works as expected.
Add support for OAuth
To add support for OAuth, we need to ensure that our Node.js code has access to the OAuth client ID and client secret.
Create a new file called .env
and add your app’s client ID and client secret. You can find these
in the OAuth tab in your app’s settings.
When we complete the OAuth flow, we’ll need a place to store the OAuth access token for each user.
Modify the code that creates the users
table as follows:
Next, we need to implement the OAuth flow itself. An OAuth flow consists of three steps:
- Redirect the user to the OAuth authorization page
- Handle the redirect back from Attio
- Exchange the authorization code for an access token
We’ll start by adding a new route to our app that redirects the user to the OAuth authorization page.
A second route will handle the redirect back from Attio.
Last, we need to ensure the user can navigate to the start of this flow. Let’s add a button to the
home page that redirects to the /integrations/attio/connect
route.
Please note, the example above stores a raw access token in the database. The access tokens that we grant to your app are highly sensitive data and should be stored securely. Please ensure any production apps you build encrypt the token before storing it.
Make a request to the Attio API and render the results
Now we have a token, all that remains is to make a request to the Attio API and render the results.
To make a request to the Attio API, we need to call the right endpoint and pass in our new oauth
token in the Authorization
header like so.
Test your app
All that remains is to spin up your app and test it out!
Run your app from the command line and visit it in your browser at http://localhost:3050
.
Was this page helpful?